coq编程
Title: Understanding Coroutines in Programming
Introduction
Coroutines have become a prevalent concept in modern programming, especially in languages like Python, Kotlin, and JavaScript. They offer a powerful way to manage asynchronous tasks and improve the efficiency of concurrent programming. In this guide, we will delve into the fundamentals of coroutines, their significance, and how they are implemented in different programming languages.
What are Coroutines?
Coroutines are a concurrency design pattern that allows for cooperative multitasking. Unlike traditional threads, which are managed by the operating system and run concurrently, coroutines are cooperatively scheduled, meaning they decide when to pause and resume execution. This cooperative nature enables efficient multitasking without the overhead of thread creation and context switching.
Key Concepts and Terminologies
1.
Coroutine
: A coroutine is a routine or function that can pause its execution and later resume from the same point. It can yield control back to the caller without blocking the entire program.2.
Coroutine Scheduler
: This is responsible for managing the execution of coroutines, deciding when to pause and resume them.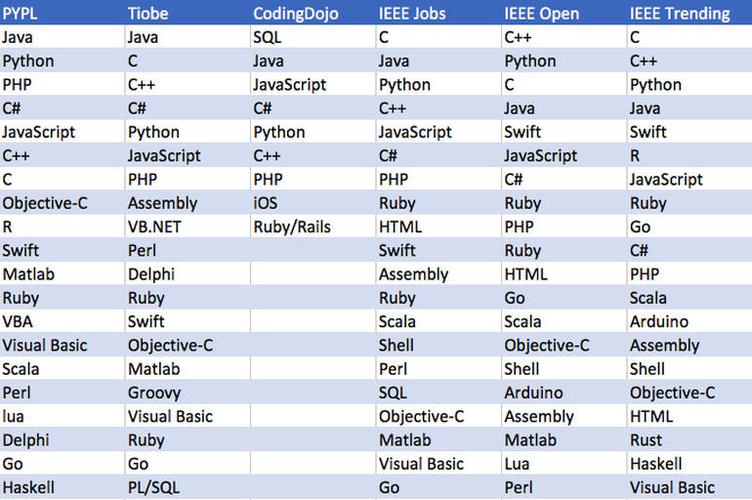
3.
Coroutine States
: Coroutines typically transition between various states, such as running, suspended, and completed.4.
Asynchronous Operations
: Coroutines are often used to handle asynchronous tasks, such as I/O operations, network requests, and user input processing.Implementations in Different Languages
1.
Python
: Python introduced native support for coroutines with the `asyncio` module. Coroutines in Python are defined using the `async` keyword, and asynchronous functions are awaited using the `await` keyword.```python
import asyncio
async def my_coroutine():
await asyncio.sleep(1)
print("Coroutine executed")
asyncio.run(my_coroutine())
```
2.
Kotlin
: Kotlin provides coroutines through the `kotlinx.coroutines` library. Coroutines in Kotlin are declared using the `suspend` modifier, and asynchronous operations are performed using `suspend` functions.```kotlin
import kotlinx.coroutines.*
suspend fun myCoroutine() {
delay(1000)
println("Coroutine executed")
}
fun main() = runBlocking {
myCoroutine()
}
```
3.
JavaScript (Node.js)
: JavaScript uses Promises and the `async/await` syntax for handling asynchronous operations. While not coroutines in the traditional sense, they achieve similar results by allowing nonblocking asynchronous execution.```javascript
async function myFunction() {
await new Promise(resolve => setTimeout(resolve, 1000));
console.log("Coroutine executed");
}
myFunction();
```
Benefits of Coroutines
1.
Efficient Resource Utilization
: Coroutines are lightweight compared to threads, making them suitable for managing large numbers of concurrent tasks without consuming excessive system resources.2.
Simplified Asynchronous Programming
: Coroutines simplify the handling of asynchronous operations, leading to more readable and maintainable code compared to traditional callbackbased approaches.3.
Improved Performance
: By avoiding the overhead of thread creation and context switching, coroutines can offer better performance, especially in I/Obound applications.4.
Enhanced Control Flow
: Coroutines provide finegrained control over the flow of execution, enabling developers to write more expressive and responsive code.Best Practices for Using Coroutines
1.
Use Asynchronous I/O
: Coroutines are particularly effective for handling I/Obound tasks, such as file I/O, network requests, and database operations.2.
Avoid Blocking Calls
: Ensure that coroutines do not perform blocking operations that can hinder the responsiveness of the application. Use asynchronous alternatives whenever possible.3.
Monitor Resource Usage
: Keep track of resource utilization, especially in applications with a high volume of concurrent coroutines, to prevent resource exhaustion and performance degradation.4.
Error Handling
: Implement robust error handling mechanisms to handle exceptions and failures gracefully, especially in asynchronous workflows where errors may occur asynchronously.Conclusion
Coroutines are a valuable tool for building responsive, scalable, and efficient concurrent applications. By understanding the principles behind coroutines and adopting best practices, developers can leverage their power to tackle complex asynchronous tasks effectively. Whether you're working in Python, Kotlin, JavaScript, or another language with coroutine support, mastering this concept can significantly enhance your programming skills and productivity.
References
:Python asyncio documentation: https://docs.python.org/3/library/asyncio.html
Kotlin coroutines documentation: https://kotlinlang.org/docs/reference/coroutinesoverview.html
Node.js documentation: https://nodejs.org/en/docs/
"Concurrency in Kotlin with Coroutines" by Nate Ebel: https://www.raywenderlich.com/11187406concurrencyinkotlinwithcoroutinesanintroduction