编程里对象是什么
ObjectOriented Programming in English
ObjectOriented Programming (OOP) is a powerful paradigm used in software development to organize code into reusable components called objects. It revolves around the concept of classes and objects, encapsulation, inheritance, and polymorphism. Let's delve into these concepts and explore their relevance in English language programming.
In OOP, a class is a blueprint for creating objects. It defines the properties (attributes) and behaviors (methods) that objects of that class will have. Think of a class as a template or a cookie cutter, and objects as the cookies you create using that cutter. In English programming, classes can represent various entities, such as nouns, actions, or concepts.
For example, consider a class named Person
. It may have attributes like name
, age
, and gender
, along with methods like introduce()
and celebrate_birthday()
. Instances of this class would represent individual people.
Encapsulation refers to the bundling of data and methods that operate on the data within a single unit, i.e., a class. This concept promotes data hiding and protects the internal state of objects from outside interference. In English programming, encapsulation can be likened to writing coherent paragraphs or essays.
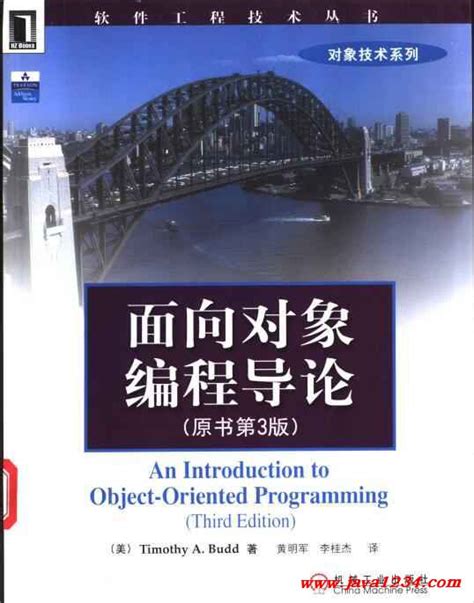
For instance, consider a class Book
. It may have attributes like title
, author
, and page_count
, along with methods like get_title()
and turn_page()
. Users of the Book
class don't need to know how these methods work internally; they only need to know how to use them.
Inheritance allows a class (subclass) to inherit properties and behaviors from another class (superclass). This facilitates code reuse and promotes a hierarchical structure. In English programming, inheritance can be compared to the relationship between a parent and child.
For example, consider a superclass Animal
with attributes like species
and habitat
. We can have subclasses like Dog
and Cat
inheriting from Animal
and adding specific attributes or methods unique to each species.
Polymorphism allows objects of different classes to be treated as objects of a common superclass. It enables flexibility and modularity in code by allowing methods to behave differently based on the object they are called on. In English programming, polymorphism can be seen in the usage of synonyms or different forms of expression.
For instance, consider a superclass Shape
with a method calculate_area()
. Subclasses like Circle
and Rectangle
can override this method to provide their own implementation, allowing the same method name to be used with different objects.
ObjectOriented Programming provides a systematic approach to software development, promoting modularity, reusability, and maintainability. In English programming, the principles of OOP can be applied metaphorically to enhance clarity, coherence, and flexibility in language use. By understanding and applying these concepts effectively, developers can write more structured and efficient code, just as proficient English speakers can communicate more effectively.