编程星星代码
Title: Creating Star Patterns with Programming
Creating star patterns using programming is a fascinating way to explore the versatility of coding. Whether you're a beginner or an experienced programmer, playing around with star patterns can enhance your understanding of loops, conditions, and algorithmic thinking. Let's delve into how you can design various star patterns using different programming languages.
1. Introduction
Star patterns are aesthetic arrangements of stars (*) in various shapes and designs. They are not only visually appealing but also serve as excellent exercises to grasp fundamental programming concepts such as loops and conditional statements.
2. Basic Star Pattern
Let's start with a simple star pattern where stars are arranged in a single line.
```python
def basic_star_pattern(rows):
for i in range(rows):
print("*", end=" ")
basic_star_pattern(5)
```
Output:
```
* * * * *
```
3. Square Star Pattern
A square star pattern consists of stars arranged in the form of a square.
```python
def square_star_pattern(rows):
for i in range(rows):
print("* " * rows)
square_star_pattern(5)
```
Output:
```
* * * * *
* * * * *
* * * * *
* * * * *
* * * * *
```
4. Right Triangle Star Pattern
In a right triangle star pattern, stars form a right triangle.
```python
def right_triangle_star_pattern(rows):
for i in range(1, rows 1):
print("* " * i)
right_triangle_star_pattern(5)
```
Output:
```
*
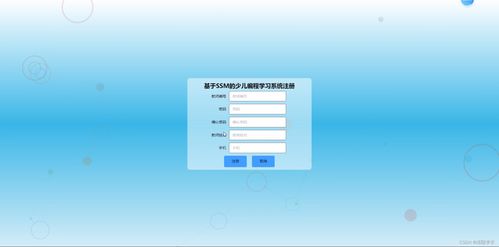
* *
* * *
* * * *
* * * * *
```
5. Inverted Right Triangle Star Pattern
This pattern is the inverse of the right triangle star pattern.
```python
def inverted_right_triangle_star_pattern(rows):
for i in range(rows, 0, 1):
print("* " * i)
inverted_right_triangle_star_pattern(5)
```
Output:
```
* * * * *
* * * *
* * *
* *
*
```
6. Diamond Star Pattern
A diamond star pattern forms a diamond shape with stars.
```python
def diamond_star_pattern(rows):
for i in range(1, rows 1):
print(" " * (rows i) "* " * i)
for i in range(rows 1, 0, 1):
print(" " * (rows i) "* " * i)
diamond_star_pattern(5)
```
Output:
```
*
* *
* * *
* * * *
* * * * *
* * * *
* * *
* *
*
```
7. Conclusion
Creating star patterns through programming not only enhances your coding skills but also boosts your logical thinking and problemsolving abilities. Experiment with different patterns, modify them, and challenge yourself to create new ones. Happy coding!
8. Further Exploration
Try implementing these patterns in other programming languages like Java, C , or JavaScript.
Experiment with nested loops to create more complex patterns.
Explore geometric patterns beyond stars, such as squares, triangles, and circles.