JAVA算法题
Title: Efficient Java Programming: Algorithmic Challenges
Java, with its robust standard library and versatile syntax, is a popular choice for implementing algorithms across various domains. Let's delve into some algorithmic challenges commonly encountered in Java programming and explore efficient solutions.
1. Sorting Algorithms:
Sorting is a fundamental operation in computer science. Java provides several builtin sorting algorithms in the `java.util.Arrays` class, such as `Arrays.sort()` which internally uses a dualpivot Quicksort for primitive types and a modified merge sort for objects. However, understanding how these algorithms work internally is crucial for optimizing performance for specific use cases.
Quick Sort:
Quick Sort is a highly efficient sorting algorithm with an average time complexity of O(n log n). In Java, you can implement it as follows:
```java
public class QuickSort {
public static void sort(int[] arr, int low, int high) {
if (low < high) {
int pivotIndex = partition(arr, low, high);
sort(arr, low, pivotIndex 1);
sort(arr, pivotIndex 1, high);
}
}
private static int partition(int[] arr, int low, int high) {
int pivot = arr[high];
int i = low 1;
for (int j = low; j < high; j ) {
if (arr[j] < pivot) {
i ;
int temp = arr[i];
arr[i] = arr[j];
arr[j] = temp;
}
}
int temp = arr[i 1];
arr[i 1] = arr[high];
arr[high] = temp;
return i 1;
}
}
```
Merge Sort:
Merge Sort is a stable sorting algorithm with a time complexity of O(n log n). It divides the array into smaller subarrays, sorts them, and then merges them back. Here's a Java implementation:
```java
public class MergeSort {
public static void sort(int[] arr, int low, int high) {
if (low < high) {
int mid = (low high) / 2;
sort(arr, low, mid);
sort(arr, mid 1, high);
merge(arr, low, mid, high);
}
}
private static void merge(int[] arr, int low, int mid, int high) {
int n1 = mid low 1;
int n2 = high mid;
int[] L = new int[n1];
int[] R = new int[n2];
System.arraycopy(arr, low, L, 0, n1);
System.arraycopy(arr, mid 1, R, 0, n2);
int i = 0, j = 0, k = low;
while (i < n1 && j < n2) {
if (L[i] <= R[j]) {
arr[k ] = L[i ];
} else {
arr[k ] = R[j ];
}
}
while (i < n1) {
arr[k ] = L[i ];
}
while (j < n2) {
arr[k ] = R[j ];
}
}
}
```
2. Graph Algorithms:
Graph algorithms are essential for solving problems involving networks, routes, and connections. Java provides implementations of various graph algorithms in the `java.util` package.
BreadthFirst Search (BFS):
BFS is a traversing algorithm that explores the neighbor nodes before moving to the next level of neighbors. It's useful for finding the shortest path in an unweighted graph.
```java
import java.util.*;
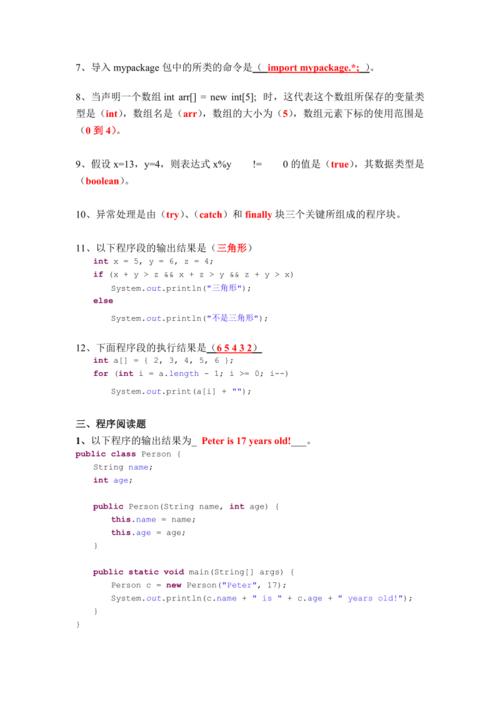
public class BFS {
public void bfs(int[][] graph, int start) {
boolean[] visited = new boolean[graph.length];
Queue
visited[start] = true;
queue.offer(start);
while (!queue.isEmpty()) {
int current = queue.poll();
System.out.print(current " ");
for (int i = 0; i < graph.length; i ) {
if (graph[current][i] == 1 && !visited[i]) {
visited[i] = true;
queue.offer(i);
}
}
}
}
}
```
DepthFirst Search (DFS):
DFS explores as far as possible along each branch before backtracking. It's useful for topological sorting, cycle detection, and solving puzzles.
```java
import java.util.*;
public class DFS {
public void dfs(int[][] graph, int start, boolean[] visited) {
visited[start] = true;
System.out.print(start " ");
for (int i = 0; i < graph.length; i ) {
if (graph[start][i] == 1 && !visited[i]) {
dfs(graph, i, visited);
}
}
}
}
```
Conclusion:
Efficient Java programming involves understanding and implementing algorithms tailored to specific use cases. By leveraging builtin libraries and writing optimized algorithms, you can tackle complex problems effectively. Remember to analyze the problem requirements and choose the most suitable algorithm to achieve optimal performance.