74hc165功能
Understanding and Programming the 74HC165 Shift Register
The 74HC165 is an 8bit parallelin, serialout shift register that allows you to expand the number of digital inputs on your microcontroller or other digital device. It's a handy integrated circuit (IC) for projects requiring more digital inputs than are available on your microcontroller.
The 74HC165 works by taking in parallel data from eight input pins and then shifting it out serially. This means you can read the state of eight different digital inputs using only a few pins on your microcontroller. It operates in a similar way to a conveyor belt, where each piece of data moves along one step at a time.
To use the 74HC165, you'll need to connect it to your microcontroller. Here's a basic wiring diagram:
74HC165 Microcontroller
_______ _______
| | | |
| A || D0 |
| B || D1 |
| C || D2 |
| D || D3 |
| E || D4 |
| F || D5 |
| G || D6 |
| /OE || D7 |
| /SR || SCK |
| /SH || RCK |
| /QH || QH' |
|_______| |_______|
- AH: These are the parallel input pins where your external digital signals will be read.
- /OE (Output Enable): When this pin is low (grounded), the output is enabled, and data can be shifted out. When it's high (connected to VCC), the output is disabled.
- /SR (Shift Register Clock): This pin controls the shifting of data into the register. Each time you pulse this pin, the next bit of data from the parallel inputs is shifted in.
- /SH (Storage Register Clock): This pin controls when the parallel input data is transferred to the storage register. When you pulse this pin, the data currently in the parallel inputs is "latched" into the shift register and is ready to be read serially.
- /QH (Serial Output): This is the serial output pin where you'll read the data from the shift register, one bit at a time.
Programming the 74HC165 involves a few simple steps:
```cpp
const int SR_CLK = 2; // Shift register clock pin
const int RCLK = 3; // Storage register clock pin
const int QH = 4; // Serial output pin
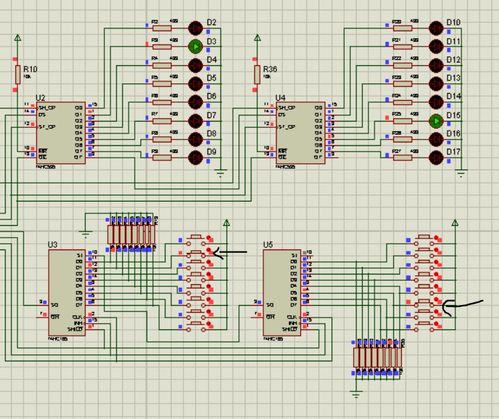
void setup() {
pinMode(SR_CLK, OUTPUT);
pinMode(RCLK, OUTPUT);
pinMode(QH, INPUT);
digitalWrite(SR_CLK, LOW);
digitalWrite(RCLK, LOW);
}
void loop() {
// Read parallel data
digitalWrite(RCLK, HIGH);
delay(1);
digitalWrite(RCLK, LOW);
// Shift out data serially
for (int i = 0; i < 8; i ) {
digitalWrite(SR_CLK, HIGH);
delay(1);
digitalWrite(SR_CLK, LOW);
// Read state of QH pin and do something with the data
Serial.print(digitalRead(QH));
}
Serial.println(); // Move to next line for next iteration
}
```
The 74HC165 shift register is a versatile IC for expanding digital input capabilities in your projects. By understanding its functionality and following a simple programming procedure, you can efficiently read multiple digital inputs using minimal pins on your microcontroller.