编程语言python入门
Title: Understanding ArrayList in Programming Languages
ArrayList is a fundamental data structure used in various programming languages, offering dynamic arrays with many functionalities. Let's delve into its concepts, implementations, and best practices across different languages.
Introduction to ArrayList:
ArrayList is a dynamic array that can resize itself dynamically as elements are added or removed. It provides flexibility and efficiency, making it a popular choice for managing collections of data.
Java:
In Java, ArrayList is part of the Java Collections Framework and resides in the java.util package. It allows storing elements of any type and provides methods for adding, removing, accessing, and manipulating elements efficiently. Here's a basic example:
```java
import java.util.ArrayList;
public class Main {
public static void main(String[] args) {
ArrayList
names.add("Alice");
names.add("Bob");
names.add("Charlie");
System.out.println(names);
}
}
```
Python:
In Python, the equivalent of ArrayList is the list data structure. Python lists are dynamic arrays by default, providing similar functionalities to Java's ArrayList. Here's a Python example:
```python
names = ["Alice", "Bob", "Charlie"]
names.append("David")
names.remove("Bob")
print(names)
```
C:
C provides the ArrayList class in the System.Collections namespace. It behaves similarly to Java's ArrayList, allowing dynamic resizing and manipulation of elements. Here's how it looks:
```csharp
using System;
using System.Collections;
class Program
{
static void Main()
{
ArrayList names = new ArrayList();
names.Add("Alice");
names.Add("Bob");
names.Add("Charlie");
names.Remove("Bob");
Console.WriteLine(string.Join(", ", names.ToArray()));
}
}
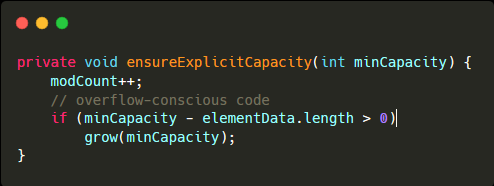
```
Common Operations:
Regardless of the language, ArrayLists support common operations such as adding elements, accessing elements by index, removing elements, and resizing dynamically.
Adding Elements:
Use `add()` in Java, `append()` in Python, and `Add()` in C.
Accessing Elements:
Employ index notation (`get()` in Java, index access in Python, direct access in C).
Removing Elements:
Use `remove()` in Java, `remove()` or `pop()` in Python, and `Remove()` in C.Best Practices:
Specify Type:
Whenever possible, specify the type of elements ArrayList will contain to ensure type safety and avoid runtime errors.
Capacity Planning:
Consider initial capacity to minimize resizing overhead, especially for large collections.
Use Generics (Java/C):
Utilize generics (ArrayList\
Prefer Resizable Arrays:
Use ArrayLists when the size of the collection is dynamic and unpredictable.Conclusion:
ArrayList is a versatile data structure available in various programming languages, offering dynamic resizing and efficient manipulation of collections. Understanding its principles and implementations across languages empowers developers to leverage its capabilities effectively in their projects.
This comprehensive guide offers insights into ArrayList across different programming languages, aiding developers in harnessing its power for efficient data management.