1.IncludeNecessaryHeaders:
Guide to Network Programming with GCC
Network programming involves writing programs that communicate over a network, such as the internet. When it comes to network programming in C, GCC (GNU Compiler Collection) is a popular choice for compiling and building network applications. Here is a guide to help you get started with network programming using GCC:
When writing network programs in C, you need to include the necessary header files. Some of the commonly used headers for network programming are:
#include#include #include #include #include #include #include
A socket is the endpoint for communication in a network. To create a socket in C, you can use the socket()
system call. Here is an example of creating a socket:
int sockfd = socket(AF_INET, SOCK_STREAM, 0); if (sockfd < 0) { perror("Error creating socket"); exit(EXIT_FAILURE); }
After creating a socket, you need to bind it to a specific port on the local machine. This is done using the bind()
system call. Here is an example of binding a socket:
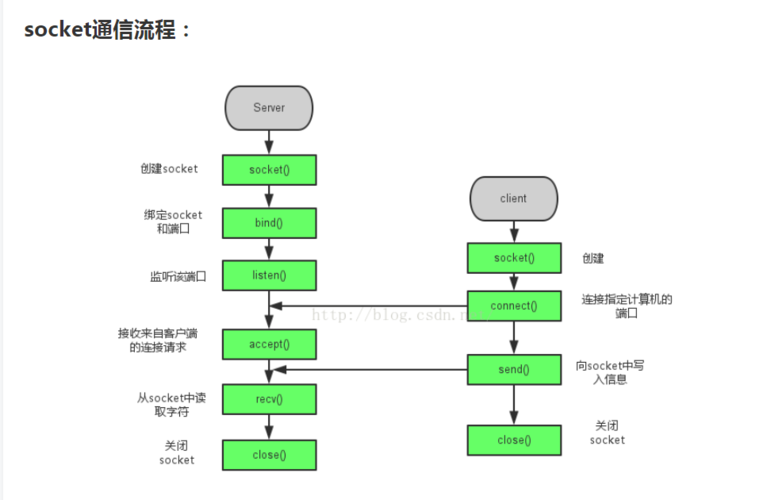
struct sockaddr_in server_address; server_address.sin_family = AF_INET; server_address.sin_addr.s_addr = INADDR_ANY; server_address.sin_port = htons(PORT); if (bind(sockfd, (struct sockaddr *)&server_address, sizeof(server_address)) < 0) { perror("Error binding socket"); exit(EXIT_FAILURE); }
If you are creating a server application, you need to listen for incoming connections and accept them. This is done using the listen()
and accept()
system calls. Here is an example:
if (listen(sockfd, BACKLOG) < 0) { perror("Error listening on socket"); exit(EXIT_FAILURE); } int new_socket = accept(sockfd, (struct sockaddr *)&client_address, &addrlen); if (new_socket < 0) { perror("Error accepting connection"); exit(EXIT_FAILURE); }
If you are creating a client application, you need to connect to a server. This is done using the connect()
system call. Here is an example:
struct sockaddr_in server_address; server_address.sin_family = AF_INET; server_address.sin_port = htons(PORT); inet_pton(AF_INET, SERVER_IP, &server_address.sin_addr); if (connect(sockfd, (struct sockaddr *)&server_address, sizeof(server_address)) < 0) { perror("Error connecting to server"); exit(EXIT_FAILURE); }
Once the connection is established, you can send and receive data using the send()
and recv()
system calls. Here is an example of sending data:
send(new_socket, message, strlen(message), 0);
And here is an example of receiving data:
char buffer[BUFFER_SIZE]; recv(new_socket, buffer, BUFFER_SIZE, 0);
After you are done with the communication, remember to close the socket using the close()
system call:
close(sockfd);
These are the basic steps involved in network programming using GCC in C. Remember to handle errors properly and always check the return values of system calls for any potential issues. Network programming can be complex, so it's important to thoroughly test your code and ensure its reliability and security.